Mastering CI/CD: Streamlining Software Development
Introduction
CI/CD, which stands for Continuous Integration and Continuous Deployment (or Continuous Delivery), is a set of best practices and tools used in software development to automate and streamline the process of building, testing, and deploying software.In very simple terms, CI is a modern software development practice in which incremental code changes are made frequently and reliably. Automated build-and-test steps triggered by CI ensure that code changes being merged into the repository are reliable. The code is then delivered quickly and seamlessly as a part of the CD process. In the software world, the CI/CD pipeline refers to the automation that enables incremental code changes from developers' desktops to be delivered quickly and reliably to production.
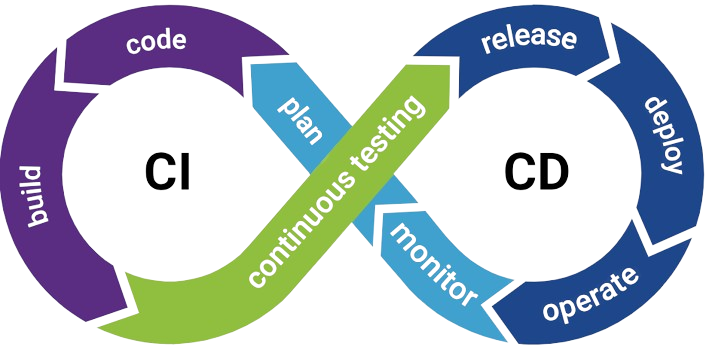
1. Continuous Integration (CI)
Objective: Integrate code changes from multiple contributors into a shared repository frequently.Key practices:
- Version Controls: Use a version control system (e.g., Git) to track changes and manage collaboration.
- Automated Builds: Set up automated build systems to compile code and generate executable artifacts.
- Automated Testing: Implement automated testing (unit tests, integration tests) to verify that the code changes do not introduce errors.
- Frequent Integration: Integrate code changes frequently into a shared repository to detect and address issues early.
2. Continuous Deployment/Delivery (CD)
Objective: Automate the process of deploying code changes to production environments.Key Practices:
- Deployment Automation: Automate the deployment process to reduce manual errors and ensure consistency.
- Continuous Deployment (CD): Automatically deploy code changes to production after passing automated tests and other quality checks.
- Continuous Delivery (CD): Prepare code changes for deployment, but the actual deployment is triggered manually.
- Environment Parity: Ensure consistency between development, testing, and production environments to avoid unexpected issues

CI/CD Pipeline
A CI/CD pipeline is a series of automated steps that code changes go through from development to production. It typically includes stages such as code compilation, automated testing, and deployment.Key Components
- Source Code Repository: A central location (e.g., Git repository) where code changes are stored.
- Build Server: Server or service responsible for compiling code and generating build artifacts
- Automated Testing Tools: Tools for running automated tests to ensure code quality.
- Artifact Repository: A repository to store compiled artifacts for deployment
- Deployment Automation: Tools and scripts to automate the deployment process.
Benefits of CI/CD
- Rapid Feedback: Early detection of bugs and issues.
- Consistent Builds: Automated builds ensure consistency across different environments.
- Faster Time to Market: Continuous deployment accelerates the release cycle.
- Reduced Manual Errors: Automation reduces the chance of human errors during deployment.
- Increased Collaboration: Continuous integration promotes collaboration among development teams.
Popular CI/CD Tools:
- Jenkins: An open-source automation server widely used for building, testing, and deploying.
- Travis CI: A cloud-based CI/CD service that integrates with GitHub repositories.
- GitLab CI/CD: Integrated CI/CD functionality within the GitLab platform.
- CircleCI: A cloud-based CI/CD platform supporting multiple programming languages.
- GitHub Actions: Native CI/CD capabilities integrated with GitHub repositories.
Software Development Methodologies
Software development methodologies play a crucial role in shaping the approach, collaboration, and efficiency of development teams. This section delves into three prominent methodologies: Waterfall, Agile, and DevOps, each with its unique principles and purposes.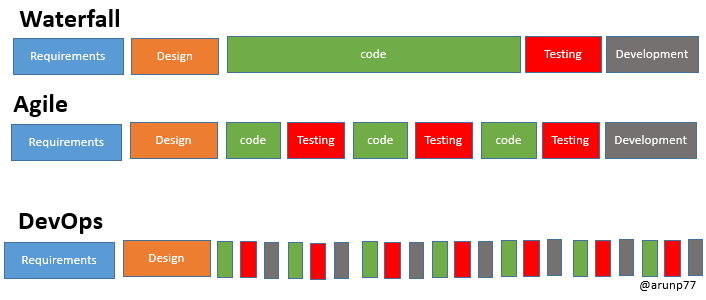
- Waterfall Methodology:
Definition: The Waterfall model is a traditional, linear approach to software development. It follows a sequential structure, with each phase building upon the previous one.
Purpose:- Phased Development: Waterfall divides the project into distinct phases - requirements, design, implementation, testing, deployment, and maintenance.
- Predictability: Provides a structured and predictable framework, making it easier to plan and manage projects.
- Minimal Client Involvement: Client involvement is limited, mainly occurring at the beginning and end of the project.
Challenges:- Rigidity: Changes are challenging to incorporate once a phase is completed.
- Delayed Feedback: Issues may not surface until the testing phase, leading to delayed feedback.
- Agile Methodology:
Definition: Agile is an iterative and incremental approach to software development, prioritizing flexibility and adaptability.
Purpose:- Iterative Development: Emphasizes the delivery of small, functional increments of the software in short iterations
- Adaptability: Welcomes changes in requirements, even late in the development process
- Customer Collaboration: Encourages constant collaboration with clients throughout the development cycle.
Challenges:- Resource Intensive: Requires close and continuous collaboration, which can be resource-intensive.
- Lack of Documentation: Limited documentation may pose challenges for larger, complex projects.
- DevOps Methodology:
Definition: DevOps is a culture and set of practices that brings together development and operations teams to streamline collaboration and automate processes.
Purpose:- Collaboration: Fosters collaboration between development, operations, and other stakeholders.
- Automation: Implements continuous integration and deployment to automate the development, testing, and deployment processes.
- Continuous Monitoring: Emphasizes continuous monitoring of applications and infrastructure for quick issue identification and resolution.
Challenges:- Cultural Shift: Requires a cultural shift and close cooperation between traditionally separate teams.
- Learning Curve: Adoption may involve a learning curve for implementing new tools and practices.
Waterfall | Agile | DevOps | |
---|---|---|---|
Adaptability | Resistant to changes after project initiation. | Embraces changes throughout the development process. | Integrates changes smoothly through automated processes. |
Customer Involvement | Limited client involvement, primarily at the beginning and end. | Constant collaboration with clients throughout development. | Focuses on collaboration between development, operations, and other stakeholders. |
Release Frequency | Releases occur at the end of the development cycle. | Regular, incremental releases. | Enables continuous delivery with frequent releases. |
Jenkins
Jenkins is an open-source automation server commonly used for building, testing, and deploying software. It supports the automation of tasks related to building, testing, and deploying code. It can be integrated with various tools and platforms, facilitating continuous integration and continuous delivery (CI/CD) pipelines.Understanding Jenkins
Jenkins, initially developed by Kohsuke Kawaguchi, is an automation server written in Java. It facilitates continuous integration and continuous delivery (CI/CD) by automating the building, testing, and deployment phases of software development.Github action for a flask application
Let's consider a hypothetical project for a web application built with Python and Flask. We'll create a GitHub Actions workflow that performs the following tasks:- Build and Test: Whenever code changes are pushed to the repository, the workflow will automatically build the application and run unit tests to ensure code quality.
- Code Analysis: After successful testing, the workflow will perform static code analysis using a linter to check for code style and potential errors.
- Deployment: If all previous steps pass successfully and changes are made to the main branch, the workflow will deploy the application to a staging environment for further testing.
.github/workflows/main.yml
) that achieves these goals:
name: CI/CD Workflow
on:
push:
branches:
- main
jobs:
build-and-test:
runs-on: ubuntu-latest
steps:
- name: Checkout Repository
uses: actions/checkout@v2
- name: Set up Python
uses: actions/setup-python@v2
with:
python-version: 3.x
- name: Install Dependencies
run: |
python -m pip install --upgrade pip
pip install -r requirements.txt
- name: Run Tests
run: pytest
- name: Code Analysis
run: flake8 .
deploy:
runs-on: ubuntu-latest
needs: build-and-test
if: github.ref == 'refs/heads/main'
steps:
- name: Deploy to Staging
# Add deployment steps here, such as deploying to a staging server or cloud platform
# Example: use SSH or API calls to deploy to a staging environment
run: echo "Deploying to staging environment"
This workflow consists of two jobs:
- build-and-test: This job is triggered on every push to any branch. It checks out the repository, sets up Python, installs dependencies, runs tests using pytest, and performs code analysis using flake8.
- deploy: This job runs only if the
build-and-test
job is successful and changes are pushed to themain
branch. It deploys the application to a staging environment.
References
Some other interesting things to know:
- Visit my website on For Data, Big Data, Data-modeling, Datawarehouse, SQL, cloud-compute.
- Visit my website on Data engineering