PostgreSQL basics
Introduction
PostgreSQL is a powerful open-source relational database management system that's widely used for data storage, retrieval, and management.
- Step 1: Installation:
- Installation on Windows:
You can visit installation Tutorials to install postgresql on windows.
- Visit the official PostgreSQL website for Windows at https://www.postgresql.org/download/windows/.
- Download the installer for your version of Windows (32-bit or 64-bit).
- Run the downloaded installer.
- Follow the installation wizard:
- Choose the installation directory.
- Select the components to install (typically include PostgreSQL Server and pgAdmin).
- Set a password for the PostgreSQL superuser (postgres).
- Choose a port number (the default is 5432).
- Select the locale and collation settings.
- Complete the installation.
- During installation, you can choose to launch Stack Builder to add additional tools and extensions.
- After installation, PostgreSQL should start automatically as a Windows service.
- You can access PostgreSQL using the command-line tool (psql) or the pgAdmin graphical interface.
- Installation on macOS:
You can install PostgreSQL on macOS using the following methods:
- Homebrew (recommended):
- Open Terminal.
- Install Homebrew if you don't have it:
/bin/bash -c "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh)"
- Install PostgreSQL:
brew install postgresql
- Start the PostgreSQL service:
brew services start postgresql
- Download from the official PostgreSQL website:
- Visit https://www.postgresql.org/download/macosx/.
- Download the PostgreSQL package for macOS.
- Double-click the downloaded file to run the installer.
- Follow the installation instructions.
You can use pgAdmin or the command-line tool (psql) to interact with PostgreSQL.
- Homebrew (recommended):
- Installation on Ubuntu (Linux):
- PostgreSQL is available in the Ubuntu repositories. You can install it using apt-get:
- Open a terminal.
- Update the package list:
sudo apt update
- Install PostgreSQL:
sudo apt install postgresql
- During the installation, a system user named 'postgres' is created.
- PostgreSQL should start automatically as a service. You can check its status with:
systemctl status postgresql
- You can switch to the 'postgres' user and use psql to access PostgreSQL:
.sudo -i -u postgres and psql
- Optionally, install the graphical interface pgAdmin by running:
sudo apt install pgadmin4
- Configure pgAdmin to connect to your PostgreSQL server.
- PostgreSQL is available in the Ubuntu repositories. You can install it using apt-get:
- Installation on Windows:
- Step 2: Basic PostgreSQL Concepts:
Understanding the basic concepts of PostgreSQL is essential for working with this relational database management system. Here are some fundamental PostgreSQL concepts
- Database: A container for storing data, isolated and independent of others.
- Table: Primary data storage objects consisting of rows and columns.
- Row: A single entry within a table, representing a specific entity or data instance.
- Column: Individual attributes or fields within a table, each with a specific data type.
- Primary Key: A column or combination of columns that uniquely identifies each row in a table.
- Foreign Key: A column or combination of columns that establishes a link between two tables.
- SQL (Structured Query Language): The language for interacting with PostgreSQL.
- Query: A SQL statement used to retrieve or manipulate data in the database.
- Schema: A logical container for organizing database objects such as tables, views, and functions.
- Data Type: Defines the kind of data that can be stored in a column.
- Index: A database object that improves query performance by creating a data structure for faster retrieval.
- Transaction: A sequence of SQL statements treated as a single unit of work for data consistency and integrity.
- Constraint: Rules on columns to enforce data integrity, e.g., NOT NULL, UNIQUE, CHECK.
- View: A virtual table created by defining a query to access and manipulate data without altering the source data.
- Backup and Restore: Backup is copying the database to prevent data loss; restore is recovering data from a backup.
- Step 3: Connecting to PostgreSQL:
To connect to PostgreSQL, you can use various tools and methods depending on your needs. Here, we'll discuss two common methods: connecting via the command line using
`psql`
, using postgresql UI interface and connecting programmatically using Python with the`psycopg2`
library.Let's explore two methods one by one.
- Connecting via Command Line (psql):
- Prerequisites: PostgreSQL should be installed on your system. You should have the necessary credentials (username, password) to connect.
- Steps:
- Open your terminal or command prompt.
- Use the
`psql`
command to initiate a connection to PostgreSQL. Replaceusername
with your PostgreSQL username anddatabase
with the name of the database you want to connect to. If you omit the database name, you'll connect to the default database with your username.
psql -U 'username' -d 'database'
- You'll be prompted to enter your password. After successful authentication, you'll be connected to the PostgreSQL server.
- You can start running SQL commands directly in the
`psql`
shell. For example:
SELECT * FROM your_table;
- To exit the psql shell, you can type:
\q
- Connecting via Command Line (psql):
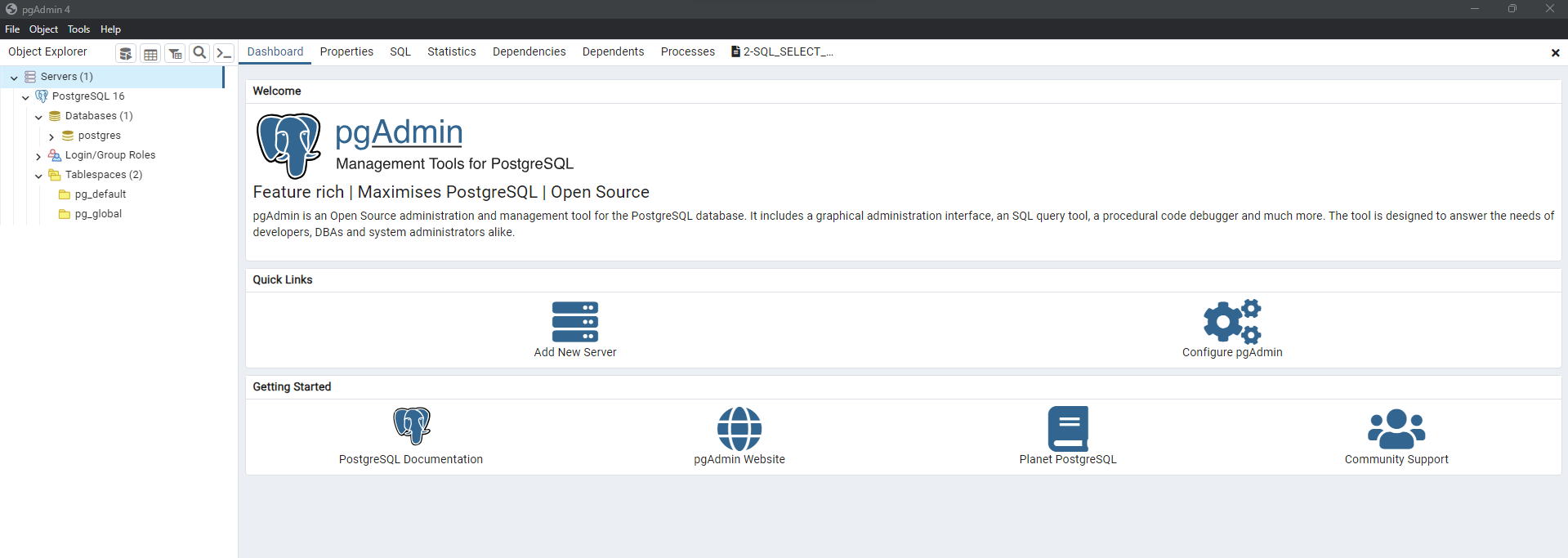
- Prerequisites:
- Python should be installed on your system.
- Install the
`psycopg2`
library, which is a PostgreSQL adapter for Python. - You can install it using
`pip`: pip install psycopg2
- Sample Python code for connecting:
import psycopg2 # Connection parameters db_params = { "host": "localhost", # Replace with your PostgreSQL server host "database": "your_db", # Replace with your database name "user": "your_user", # Replace with your PostgreSQL username "password": "your_password" # Replace with your PostgreSQL password } # Establish a connection conn = psycopg2.connect(**db_params) # Create a cursor cur = conn.cursor() # Execute SQL queries cur.execute("SELECT * FROM your_table") result = cur.fetchall() for row in result: print(row) # Close the cursor and connection cur.close() conn.close()
Creating databases and tables in PostgreSQL is a fundamental task when setting up a new project or working with an existing database.
- Creating a Database:
CREATE DATABASE your_database_name;
- Connecting to the New Database:
After creating the database, you can connect to it using
`psql`
or your preferred database client. For example, if you're using`psql`
, you can connect as follows:psql -U your_username -d your_database_name
- Creating Tables in a Database:
CREATE TABLE your_table_name ( column1_name data_type1, column2_name data_type2, ... );
Example:
CREATE TABLE users ( id serial PRIMARY KEY, username VARCHAR(50) NOT NULL );
- Creating Tables with Constraints:
CREATE TABLE products ( product_id serial PRIMARY KEY, product_name VARCHAR(100) NOT NULL, price DECIMAL(10, 2) NOT NULL, unique (product_name) );
- >Adding Data to Tables:
INSERT INTO users (username) VALUES ('john_doe');
- >Viewing Table Data:
SELECT * FROM users;
Reference:
- Visit the "Learn about PostgreSQL"
- Visit the "DBMS Tutorials page"
- Visit my "Github repository on SQL" to learn about basics and some example projects.
- Visit my "Github repository" to learn about databases.